First, create a new window service project.
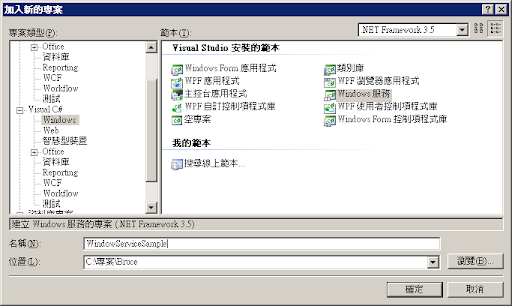
For schedule service, we need to add
System.Timers.Timer to toolbox, and drag it into design mode.
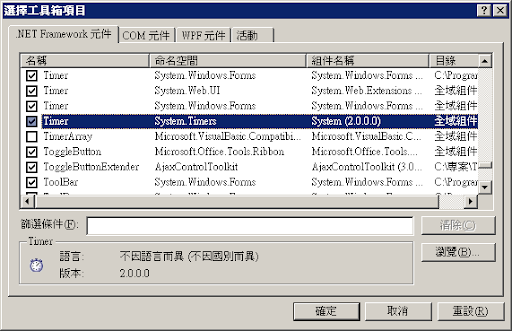
Then, code something.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Diagnostics;
using System.Linq;
using System.ServiceProcess;
using System.Text;
using System.IO;
namespace WindowServiceSample
{
public partial class Service1 : ServiceBase
{
public Service1()
{
InitializeComponent();
}
protected override void OnStart(string[] args)
{
// 服務啟動時執行
this.EventLog.WriteEntry(System.AppDomain.CurrentDomain.BaseDirectory); // write current directory to event log
}
protected override void OnStop()
{
// 服務結束時執行
}
private void timer1_Elapsed(object sender, System.Timers.ElapsedEventArgs e)
{
// 計時器, 依據設定間隔執行
}
}
}
After coding, we need to add a installer for Window Service Installer.
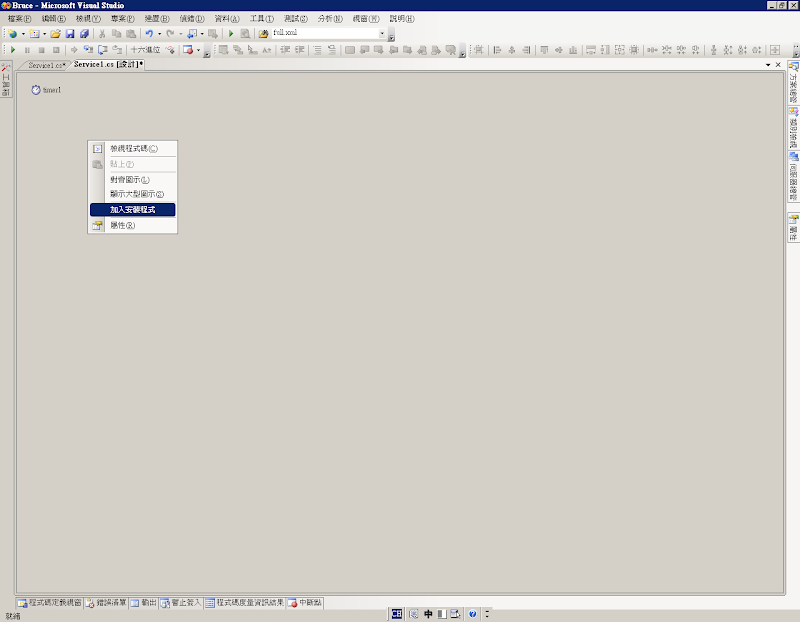
There are two components needed to set,
serviceProcessInstaller and
serviceInstaller
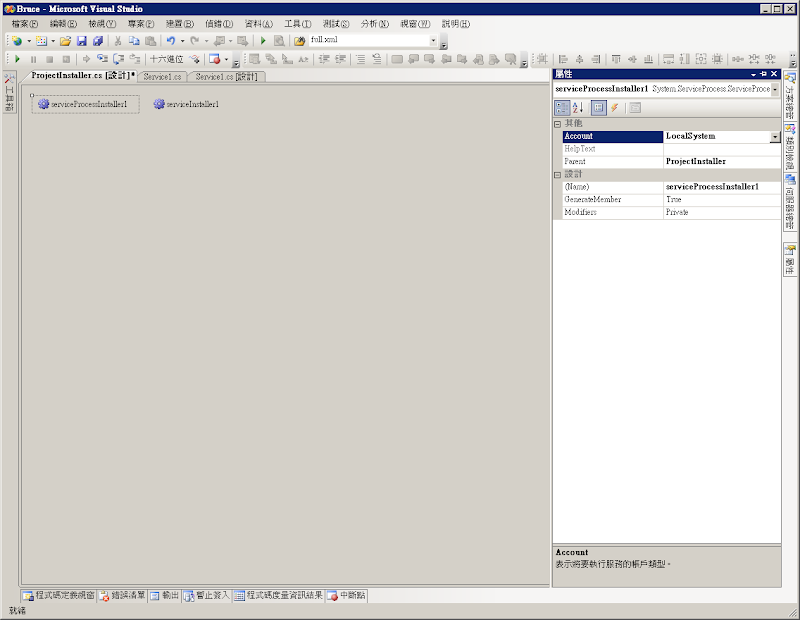
Build the project and find the executable binary, enter the command below for installing.
%windir%\Microsoft.NET\Framework\v2.0.50727\installutil WindowServiceSample.exe
There will be a new service listed in Services.
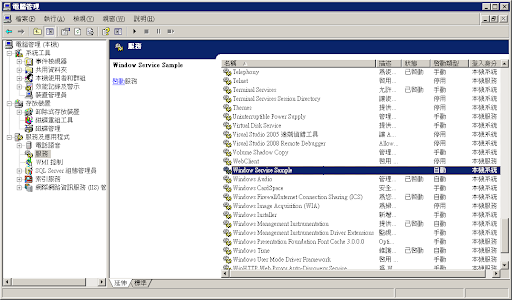
For unstalling, enter command below:
%windir%\Microsoft.NET\Framework\v2.0.50727\installutil /u WindowServiceSample.exe