Prepare a binary output action for file output
[RoutePrefix("images")] public class ImageController : Controller { // GET: ~/images/profile.png // GET: ~/images/1873429732.jpg [HttpGet] [Route("{name}.{ext}")] public ActionResult ReadImage(string name, string ext) { if (System.IO.File.Exists(Server.MapPath(string.Format("~/uploads/{0}.{1}", name, ext)))) { byte[] data = System.IO.File.ReadAllBytes(Server.MapPath(string.Format("~/uploads/{0}.{1}", name, ext))); string contentType = MimeMapping.GetMimeMapping(System.IO.Path.GetFileName(string.Format("{0}.{1}", name, ext))); return File(data, contentType); } else { return new HttpStatusCodeResult(404); } } }
Then try test it, but respone 404 not found.
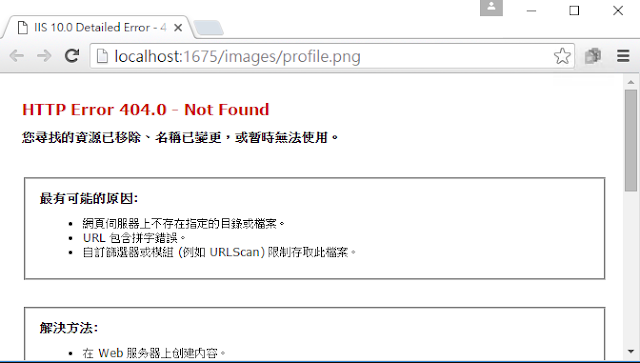
Add runAllManagedModulesForAllRequests="true" on modules section.
<?xml version="1.0" encoding="utf-8"?> <configuration> ... <system.webServer> ... <modules runAllManagedModulesForAllRequests="true"> <remove name="ApplicationInsightsWebTracking"/> <add name="ApplicationInsightsWebTracking" type="Microsoft.ApplicationInsights.Web.ApplicationInsightsHttpModule, Microsoft.AI.Web" preCondition="managedHandler"/> </modules> </system.webServer> ... </configuration>
Then try it again, image rendered success.
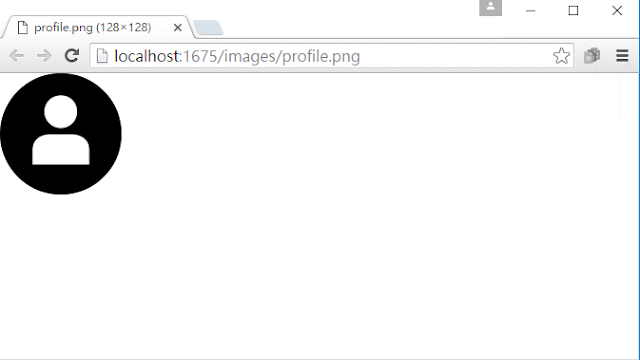